C# (CSharp) - The Basics of a Project
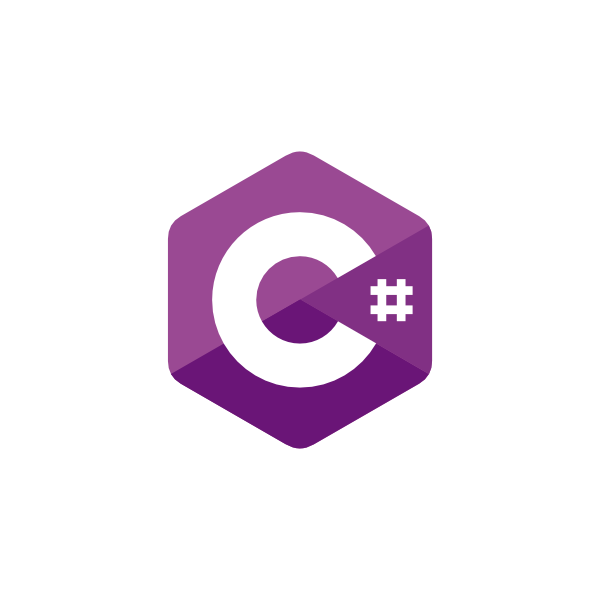
Hello koalas
This post is about some basic's parts of a project in C# with Visual Studio.
Table of Contents
AssemblyInfo.cs
The AssemblyInfo.cs file gives information about your application. You can write a description for your application, give a copyright, and indicate the current version of your application.
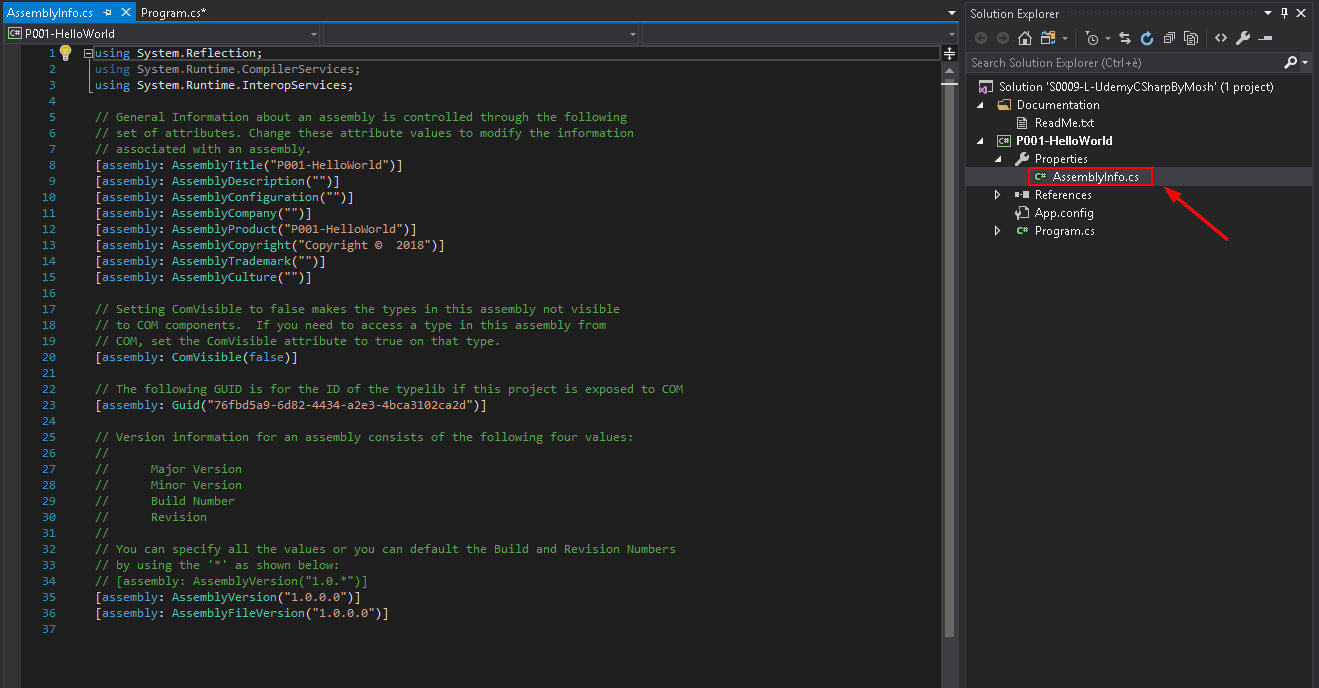
References
The references section shows you what are the references linked to your project.
A reference is a link to a class library which contains some code available to use into your application. Such as the class library "System" which contains fundamental classes and base classes that define commonly used value and reference data types, events and event handlers, interfaces, attributes, and processing exceptions.
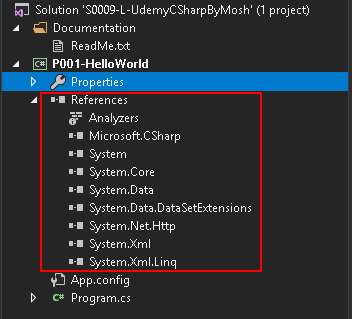
App.config
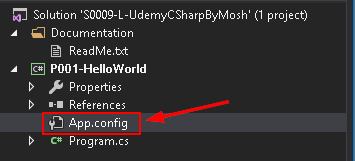
Source: Stackoverflow
At its simplest, the "app.config" is an XML file with many predefined configuration sections available and support for custom configuration sections. A "configuration section" is a snippet of XML with a schema meant to store some type of information.
Settings can be configured using built-in configuration sections such as connectionStrings or appSettings. You can add your own custom configuration sections.
Web applications typically have a "web.config", while Windows GUI/service applications have an "app.config" file.
Info: application-level config files inherit settings from global configuration files, e.g., the machine.config.
Reading from the App.Config
Connection strings have a predefined schema that you can use. Note that this small snippet is actually a valid "app.config" (or web.config) file:
<?xml version="1.0"?>
<configuration>
<connectionStrings>
<add name="MyKey"
connectionString="Data Source=localhost;Initial Catalog=ABC;"
providerName="System.Data.SqlClient"/>
</connectionStrings>
</configuration>
Once you have defined your "app.config", you can read it in code using the ConfigurationManager class.
string connectionString = ConfigurationManager.ConnectionStrings["MyKey"].ConnectionString;
Writing to the App.Config
Frequently changing the *.config files is usually not a good idea, but it sounds like you only want to perform one-time setup.
Suppose you manually change some <value> in app.config, save it and then close it.
Now when you go to your bin folder and launch the .exe file from here, why doesn't it reflect the applied changes?
The reason is that when you compile an application, its app.config is copied to the bin directory with a name that matches your executable file (.exe).
For example, if your exe was named "test.exe", there should be a "test.exe.config" in your bin directory.
You can change the configuration without a recompile, but you will need to edit the config file that was created at compile time, not the original app.config.
Source 2 : how to use app config
Add a reference to System.Configuration and include it using the using statement in your code:
using System.Configuration;
Edit "app.config" so that you add your settings in the appSettings node in an add element with key and value parameters.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.5" />
</startup>
<appSettings>
<add key="server" value="localhost" />
<add key="port" value="5432" />
<add key="username" value="myusername" />
<add key="password" value="mypass" />
<add key="database" value="mydb" />
</appSettings>
</configuration>
Now you can access your settings in code like this:
string server = ConfigurationManager.AppSettings["server"].ToString();
string port = ConfigurationManager.AppSettings["port"].ToString();
string username = ConfigurationManager.AppSettings["username"].ToString();
string password = ConfigurationManager.AppSettings["password"].ToString();
string database = ConfigurationManager.AppSettings["database"].ToString();
Program.cs
The Program.cs file contains your code.
A "void" method means "nothing". The method doesn't return anything in output.
Below we can see the line "using System;" is now active. It's because "Console" is used in the code which belong to this class.
You can get rid of the unused classes by pressing "Ctrl + ." when you are on one of these "grey" (inactive) lines and then press "Enter". Visual Studio Editor will delete the unused code for you.
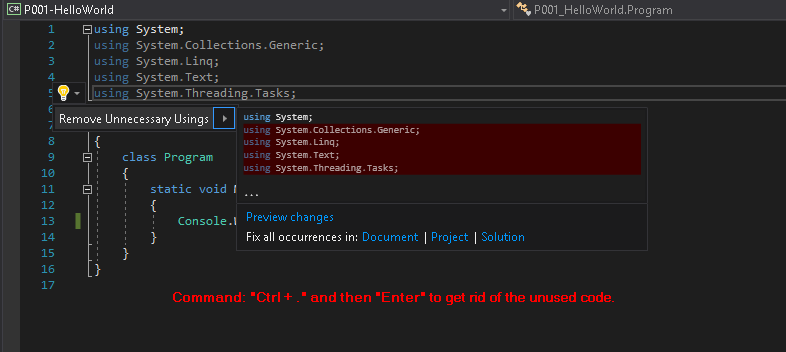
Have a nice time after!
Didier