C# (Csharp) - csc.exe, the C# compiler
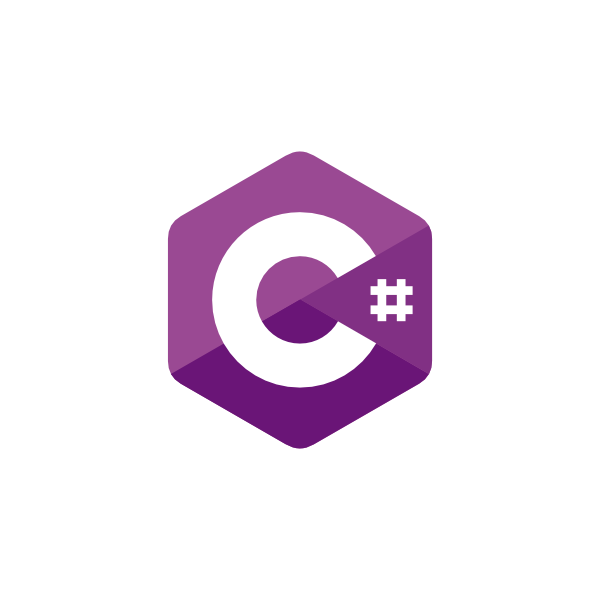
Hello koalas,
Here is my demo how to create a wonderful application to demonstrate how the C# compiler (csc.exe) works.
Table of Contents
Create folder and files
Firtly, create a folder called "MyApp".
Then create 2 files: Program.cs and MyLibrary.cs
Add this code to each file:
Program.cs:
using System;
using MyLibrary;
namespace AppByHand
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
// Use of my library "Library.cs"
Car car = new();
Console.WriteLine(car.Start());
Console.WriteLine(car.Stop());
Console.ReadLine();
}
}
}
MyLibrary.cs:
namespace MyLibrary
{
public class Car
{
public string Start()
{
return "My car starts.";
}
public string Stop()
{
return "My car stops.";
}
}
}
Check access to csc.exe
Then open a shell (cmd, PowerShell, or other) and verify that you have access to the C# compiler by typing: csc.exe -version
An example of what you should have before to start compiling your application:
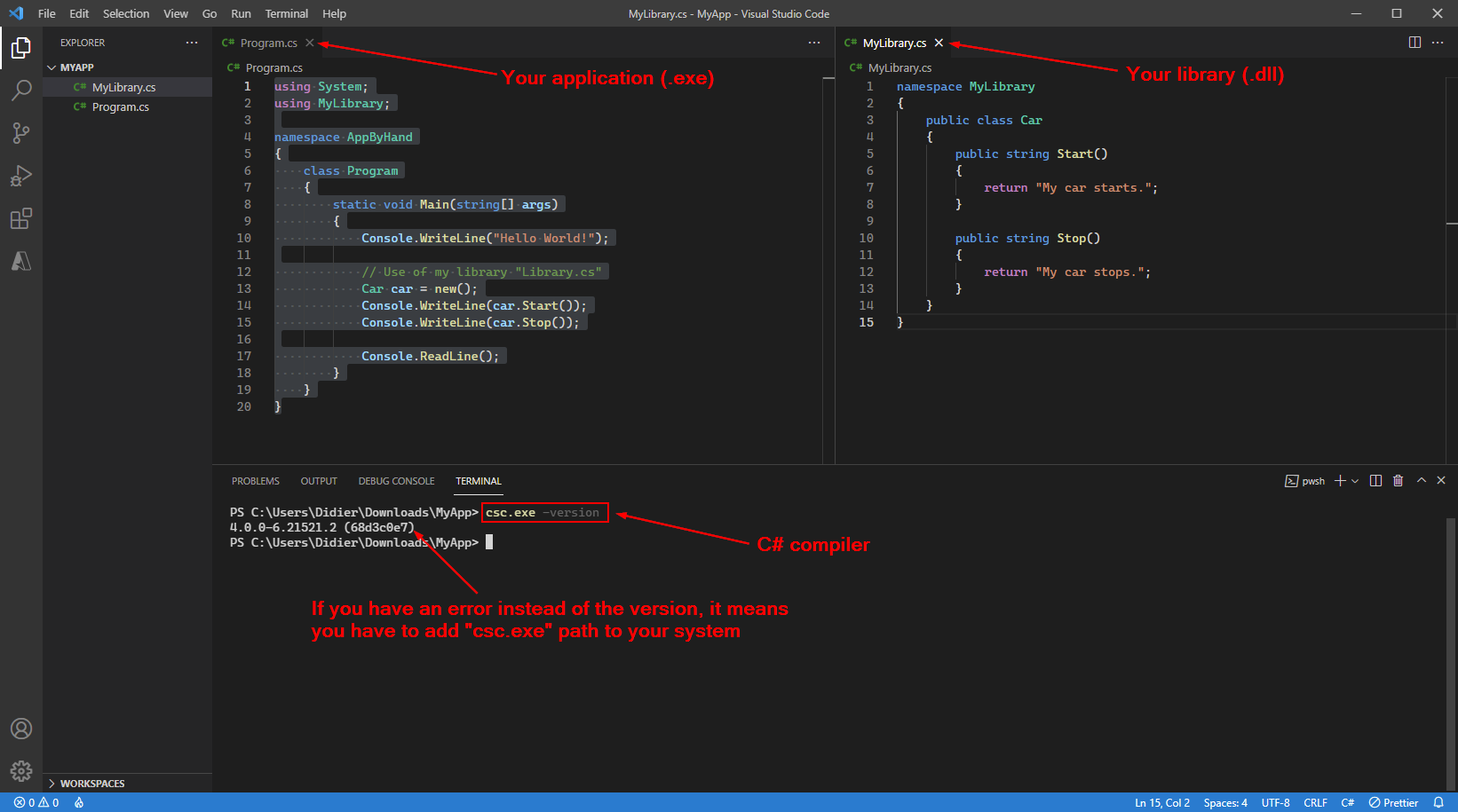
If you have no access to csc.exe command you have to add it to your system variable.
Firstly, you have to find out where your csc.exe compiler is.
It has 2 places to find it out but be careful because it's not the same one!
The C# compiler include with your Windows is at:
"C:\windows\Microsoft.NET\Framework64\[Version]\csc.exe"
Per example:
Version: v4.0.30319
Information: "csc.exe -version" command returns version 4.8.xyz. This version is include in .NET Framework 4.8 that is a part of Windows by default.
The C# compiler include with Visual Studio is at:
"C:\Program Files\Microsoft Visual Studio\[Edition]\[Your Version]\MSBuild\Current\Bin\Roslyn\csc.exe"
Per example:
Edition = 2017, 2019, or 2022
Your version = Preview, Community, Professional, or Enterprise
Information: "csc.exe -version" command returns version 4.0.xyz. This version is include in Visual Studio 2022 and is NOT a part of Windows by default.
Now, add the path of your compiler (csc.exe) to your "Environment variables":
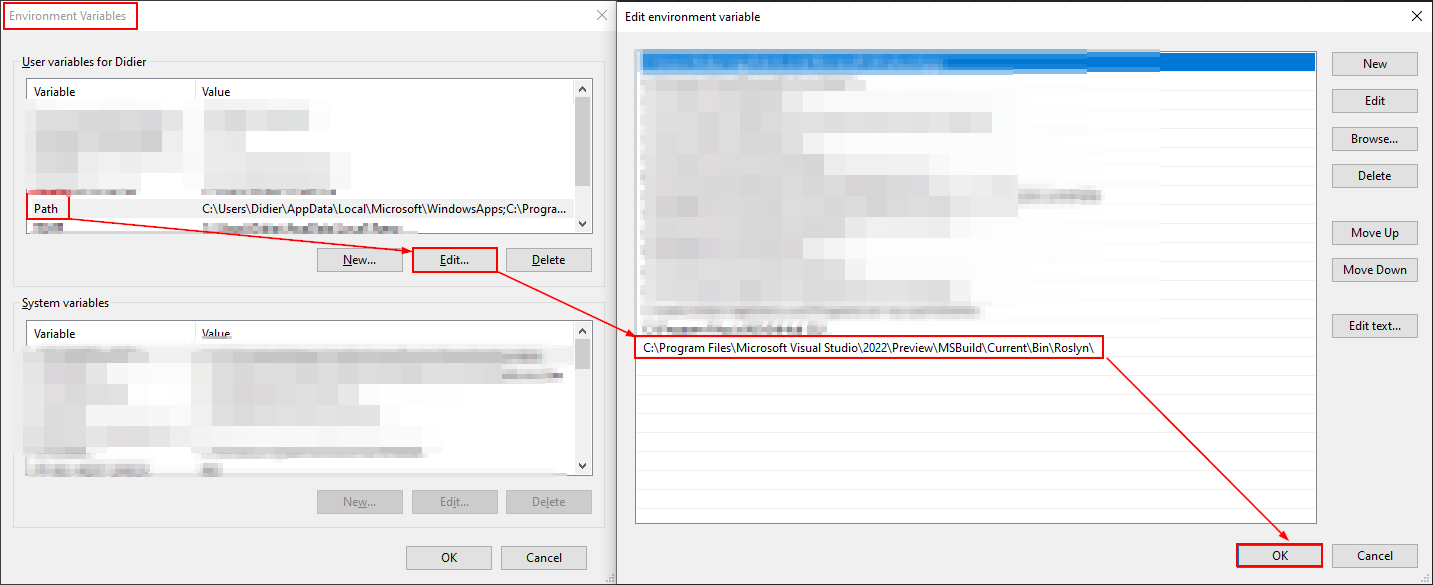
Check everything
Now, you have:
- Your application "entry point" (Program.cs)
- Your library, full of methods 😀 (MyLibrary.cs)
- Access to the C# compiler (csc.exe)
You are ready to start compiling your application (MyApp.exe).
Compiling your application
You have to type 2 commands to compile your application:
- A command to compile your library first
- A command to compile your application and link the library to it
Here are the commands separated by a semi-colon(;):
csc.exe .\MyLibrary.cs /target:library /out:MyLibrary.dll;csc.exe .\Program.cs /target:exe /out:MyApp.exe /r:MyLibrary.dll

Result
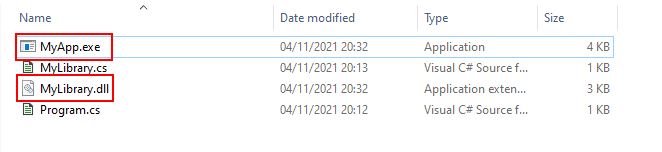
If you run your application (MyApp.exe), you should get this:
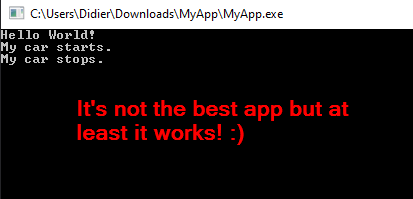
I hope you enjoyed this article.
Didier
Sources used
Name | Link |
Working with the C# 2.0 Command Line Compiler | Link |
Miscellaneous C# Compiler Options | Link |